This React and Greensock Tutorial is focusing mainly on the basics of targeting elements and using React Hooks with GreenSock.
If you have been following my blog and YouTube channel for a while, you know that I enjoy using React and GreenSock.
They are both very powerful libraries with elegant APIs.
As more and more websites are build using React, it’s important to know how to use GreenSock in the React world.
What will you learn:
- How to include GSAP in your React Project
- How to target elements using refs
- How to animate React ref using GSAP
- How to animate when React state changes
- How to create an array of refs
- How to use ScrollTrigger with React
Baby steps I know, but lets learn how to walk before you try to run.
If you prefer learning by watching videos, checkout React + GreenSock 101.
1. How to include GSAP in your React Project
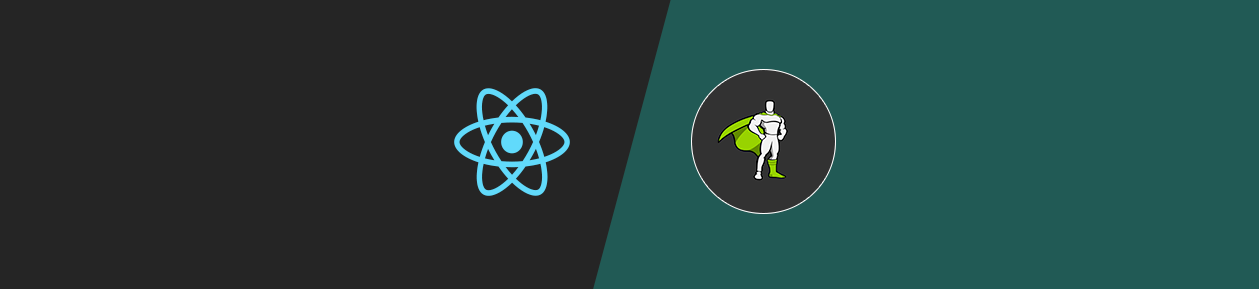
I am assuming you already know how to spin up a brand new React project using the Create React App.
npx create-react-app gsap-app cd gsap-app
Now we can install GreenSock through npm and start the app.
npm i gsap npm start
Cool, GSAP is installed lets import it to our App
component.
import React from 'react'; import { gsap } from "gsap"; const App = () => { ... return ( <div className="App"> <header className="App-header"> <img src={logo} className="App-logo" alt="logo" /> </header> </div> ) }
2. How to target elements using refs
Firstly we need to get access to the element that we want to animate.
We can use useRef
because our App
component is a functional component.
import React, { useRef } from 'react'; import { gsap } from "gsap"; const App = () => { const headerRef = useRef(null); ... return ( <div className="App"> <header ref={headerRef} className="App-header"> <img src={logo} className="App-logo" alt="logo" /> </header> </div> ) }
We are storing a reference to our header
element in the headerRef
variable.
You can read more about React Refs in the official documentation.
3. How to animate React ref using GSAP
With our header
“selected”, we can create a tween that will play when the component mounts.
import React, { useRef, useEffect } from 'react'; ... const App = () => { ... useEffect(() => { gsap.from(headerRef.current, { autoAlpha: 0, ease: 'none', delay: 1 }); }, []); ... }
Firstly we need to import useEffect
and create our tween inside of it.
The empty array as a dependency of this effect will make sure that our tween runs only on the initial mount
of this component.
If you are new to React Hooks you can check my free online course React 101.
The target for our .fromTo tween is headerRef.current
that is how we can access the underlying DOM element.
Ok, now we have our header fading in with a slight delay, but how do we animate when the state of our component changes?
4. How to animate when React state changes
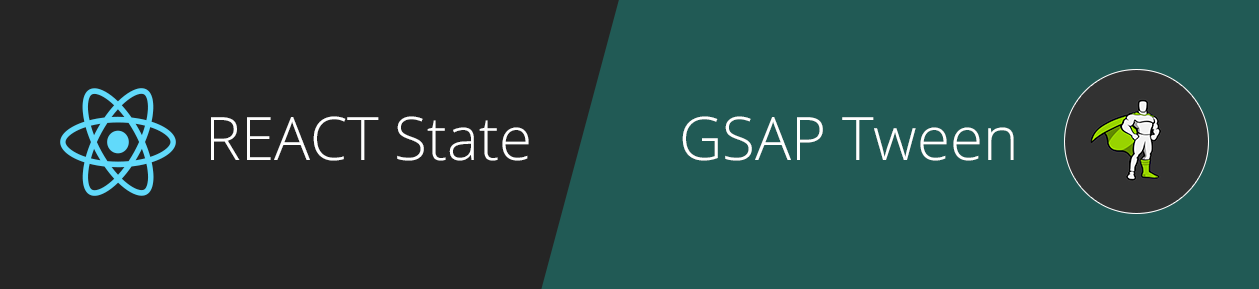
Lets create a button that will change the background color of the header
.
But we could that with css
class, right? Off course we could, but I thought you are here to learn React + Greensock.
import React, { useRef, useEffect, useState } from 'react'; ... const App = () => { const [background, setBackground] = useState('#5a7d95'); ... const toggleBackground = () => { const color = background !== '#5a7d95' ? '#5a7d95' : '#1b4943'; setBackground(color); } useEffect(() => { gsap.to(headerRef.current, { backgroundColor: background, duration: 1, ease: 'none' }); }, [background]); return ( <div className="App"> ... <button onClick={() => toggleBackground()}>Change background</button> ... </div> ); }
We import useState
, set the default color and create a function toggleBackground
that will toggle the background color between light and dark.
Then inside of a new useEffect
that only listens to the background color change, we are using GreenSock’s .to
tween to animate the background-color to the right value.
Please excuse my non-creative animations today, I am trying to keep the example as simple as possible.
5. How to create an array of refs
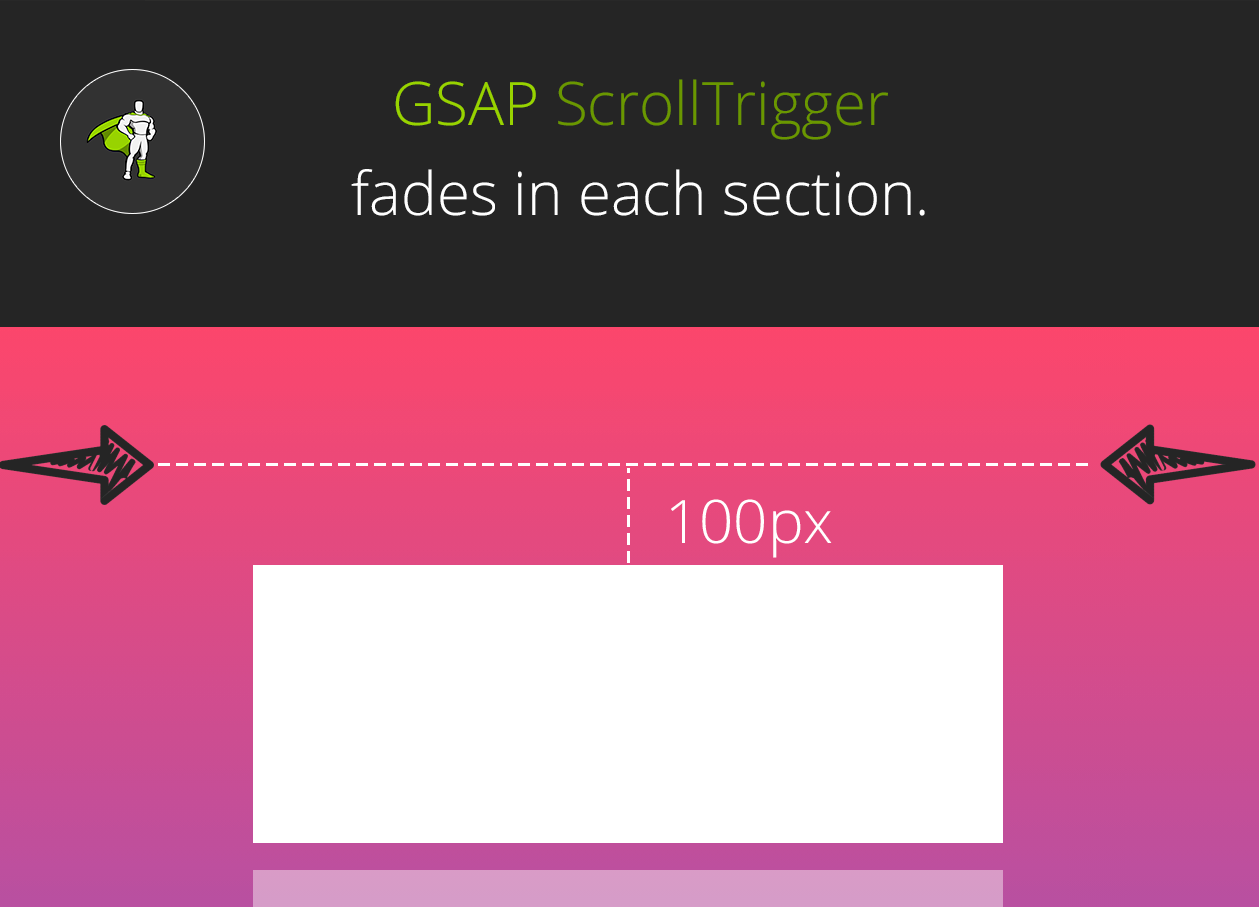
ScrollTrigger is the cool plugin from GreenSock that lets you trigger animations as the user scrolls through your page.
Let have a look how to include ScrollTrigger in your React project and fade in a few section.
import React, { useRef, useEffect, useState } from 'react'; import { ScrollTrigger } from 'gsap/ScrollTrigger'; gsap.registerPlugin(ScrollTrigger); const sections = [ { title: 'Title 1', subtitle: 'Subtitle 1' }, { title: 'Title 2', subtitle: 'Subtitle 2' }, { title: 'Title 3', subtitle: 'Subtitle 3' } ]; ...
We import ScrollTrigger and then register the plugin to make sure it survives the code tree shaking.
For this demo we are hardcoding some content in the sections
array.
const App = () => { ... const revealRefs = useRef([]); revealRefs.current = []; const addToRefs = el => { if (el && !revealRefs.current.includes(el)) { revealRefs.current.push(el); } }; return ( <div className="App"> { sections.map(({title, subtitle}) => ( <div className="App-section" key={title} ref={addToRefs}> <h2>{title}</h2> <p>{subtitle}</p> </div> )) } </div> ); }
Because we want to access multiple sections and trigger them individually, we need to create an array of refs revealRefs
.
As you know from the above header
example we get access to the underlying DOM elements in the .current
property.
That is why we need to create a function addToRefs
and add all our references to the sections to the revealRefs.current
array.
6. How to use ScrollTrigger with React
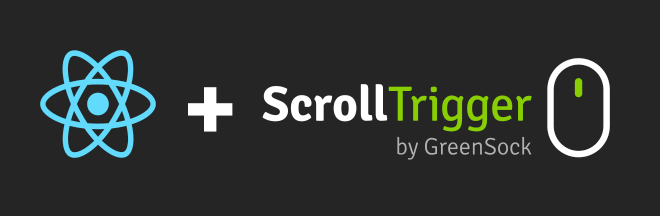
Ok, great. We have access to all sections. Now lets loop over them and create new GSAP tween with scrollTrigger
.
useEffect(() => { ... revealRefs.current.forEach((el, index) => { gsap.fromTo(el, { autoAlpha: 0 }, { duration: 1, autoAlpha: 1, ease: 'none', scrollTrigger: { id: `section-${index+1}`, trigger: el, start: 'top center+=100', toggleActions: 'play none none reverse' } }); }); }, []);
We are looping over the revealRefs.current
array and creating a simple .fromTo tween.
Each sections fades in when top of it is 100 pixels from the center of the viewport.
Again, today I could not think of a more creative way than just simply fading in a bunch of dummy sections.
React and GreenSock Tutorial Demo
Do you want to learn more about React or GreenSock? Check out my free online courses React 101 and GreenSock 101.
Conclusion
Quite a long tutorial, but I hope that this guide will help you with implementing GreenSock in your React projects.
Do you have any questions related to GSAP and React? Let me know in the comments.
very interesting tutorial 🙂 looking forward to the full course;)
Thanks, Jonathan, I thought people don’t leave comments on blog posts anymore 🙂 Great to hear that you found it useful.
gsap ScrollTrigger with create-react-app doesn’t look to work. 🙁