Are you building a one page parallax scrolling website and getting concerned about your page load time?
Lets be honest, the one page websites can get quite bulky very quickly even when you optimize everything.
You might be also interested in How To Make Page Transitions in HTML – Barba.js and GSAP3 Tutorial
Related Screencast
One option to reduce the frustration of your users is to add a nice custom preloader screen.
In this tutorial we will add a CSS3 transitions to our already created CSS3 preloader.
Once the content of the page is loaded, we’ll animate the preloading screen away from the viewport with a nice transition.
What you will learn
- how to use
CSS3 transitions
- how to
animate out
a full-screen preloader - how to combine
CSS3
withJavaScript
for this technique
Open the starting files and follow the steps below.
1. Add HTML and CSS for loading overlay
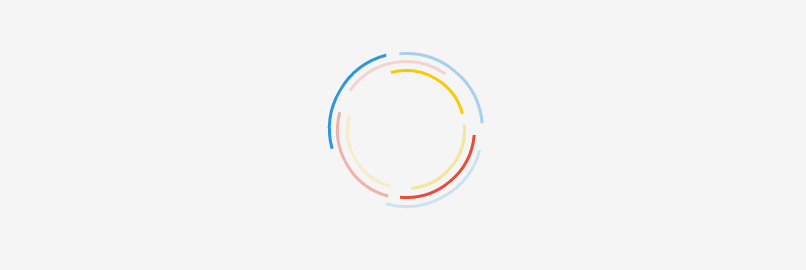
In our index.html
is an existing CSS3 preloader #loader
on a white background, but we want to create a high contrast between preload screen and the content.
Lets add two parts of the preloading screen inside of #loader-wrapper
.
<div id="loader-wrapper"> <div id="loader"></div> <div class="loader-section section-left"></div> <div class="loader-section section-right"></div> </div>
Now we’ll add some CSS into the css/main.css
, just after the @keyframes spin
declaration.
#loader-wrapper .loader-section { position: fixed; top: 0; width: 51%; height: 100%; background: #222222; z-index: 1000; } #loader-wrapper .loader-section.section-left { left: 0; } #loader-wrapper .loader-section.section-right { right: 0; }
This creates a full screen dark overlay, but unfortunately it also covers our rotating #loader
and makes the page title hard to read.
CSS Tweaks
We will need to tweak the z-index
of #loader
to bring it above our dark overlay and change the color of our heading to light grey.
Find #loader
in the stylesheet (line 43) and add the following style, also include the h1
style just below our default p
styles (line 34).
#loader { z-index: 1001; /* anything higher than z-index: 1000 of .loader-section */ } h1 { color: #EEEEEE; }
Cool, this is looking much better.
2. Add and style our default content
Now lets add some default content to the page so we have something to reveal.
Include a new div #content
just below the closing #loader-wrapper
tag.
HTML
<div id="content"> <h2>This is our page title</h2> <p>Lorem ipsum dolor sit amet.</p> </div>
As you can see this content is hidden behind our preloader screen so lets comment out the #loader-wrapper .loader-section
for a while and style our content.
Add the following into the main.css
.
#content { margin: 0 auto; padding-bottom: 50px; width: 80%; max-width: 978px; }
We are simply centering our content container and setting a width
and padding-bottom
.
And now to the fun part of animating the two preloading screen sections out of the view.
3. Add preloader transition
We will move both parts out of the view using CSS3 transforms. When the body gets a class .loaded
.
Add the following code to main.css
.
/* Loaded */ .loaded #loader-wrapper .loader-section.section-left { -webkit-transform: translateX(-100%); /* Chrome, Opera 15+, Safari 3.1+ */ -ms-transform: translateX(-100%); /* IE 9 */ transform: translateX(-100%); /* Firefox 16+, IE 10+, Opera */ } .loaded #loader-wrapper .loader-section.section-right { -webkit-transform: translateX(100%); /* Chrome, Opera 15+, Safari 3.1+ */ -ms-transform: translateX(100%); /* IE 9 */ transform: translateX(100%); /* Firefox 16+, IE 10+, Opera */ }
We’ll also fade the preloader out at the same time, lets add this to our stylesheet.
.loaded #loader { opacity: 0; }
Changing the #loader
opacity to 0 seems to be working but that will make our #content
not accessible, because the #loader
container is still sitting on top of our content.
We need to add visibility: hidden;
to #loader-wrapper
.
.loaded #loader-wrapper { visibility: hidden; }
To preview our styles we will need to manually add the .loaded
class to the body
element.
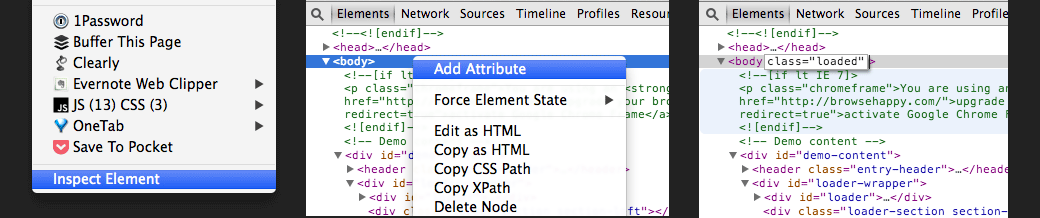
In Google Chrome right click anywhere on the page and click on inspect element, this will bring up the developer tools.
Right click on the body element and add a new attribute class="loaded"
.
Hit enter and you’ll see our preloader screen disappear.
Great, but hang on, where are the promised CSS3 transitions?
Lets do them in the next step.
4. Order of the animations
Firstly lets get the theory of our transition right.
The order of our animations should be:
- fade
#preloader
out - animate both left and right side of the overlay out of the view
- animate the whole
#loader-wrapper
out of the view
We will fade out the preloader first, by adding CSS3 transitions to #loader
.
Tip: you can get the necessary CSS3 code snippet with all the required vendor prefixes from css3please.com.
.loaded #loader { opacity: 0; -webkit-transition: all 0.3s ease-out; transition: all 0.3s ease-out; }
Then we will animate both sides out of a view with a slight 0.3s delay.
.loaded #loader-wrapper .loader-section.section-right, .loaded #loader-wrapper .loader-section.section-left { -webkit-transition: all 0.3s 0.3s ease-out; transition: all 0.3s 0.3s ease-out; }
The first 0.3s
defines animation duration and the second 0.3s
defines the animations delay.
And lastly we will animate the whole #loader-wrapper
out of the view using transform: translateY(-100%)
.
.loaded #loader-wrapper { -webkit-transform: translateY(-100%); -ms-transform: translateY(-100%); transform: translateY(-100%); -webkit-transition: all 0.3s 0.6s ease-out; transition: all 0.3s 0.6s ease-out; }
As you can see, here we are setting the delay to 0.6s
which is the total duration of our first two animations (0.3 + 0.3 = 0.6
).
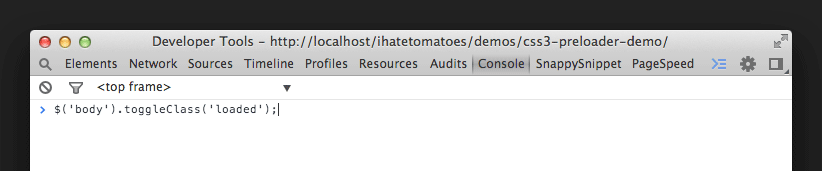
Another way to quickly preview the body class .loaded
is to run this line of jQuery
code in the web developer console.
$('body').toggleClass('loaded');
This will toggle between our content and the preloader screen animating out.
Pretty cool, huh?
5. Fine tuning
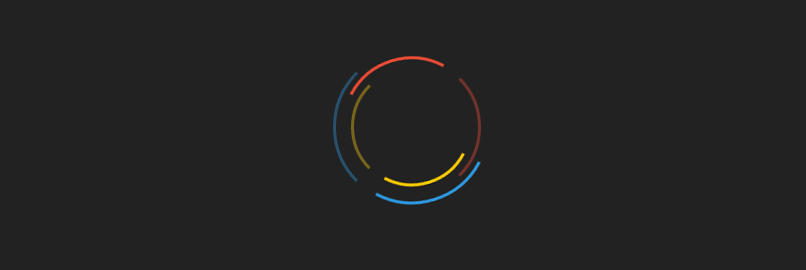
To get even slicker animation for the left and right overlay we can change the easing from ease-out
to cubic-bezier
and adjust the timing to 0.7s.
.loaded #loader-wrapper .loader-section.section-right, .loaded #loader-wrapper .loader-section.section-left { -webkit-transition: all 0.7s 0.3s cubic-bezier(0.645, 0.045, 0.355, 1.000); transition: all 0.7s 0.3s cubic-bezier(0.645, 0.045, 0.355, 1.000); }
Changing the duration of left and right section transition also means that we have to adjust the delay on .loaded #loader-wrapper
animation to 1s.
.loaded #loader-wrapper { -webkit-transform: translateY(-100%); -ms-transform: translateY(-100%); transform: translateY(-100%); -webkit-transition: all 0.3s 1s ease-out; transition: all 0.3s 1s ease-out; }
6. Fake it till you make it
Because we don’t have enough “heavy” content on our page, we will be faking the loading of the content with a simple jQuery.
You can use imagesloaded by David DeSandro or PxLoader by Pixel Lab on your own projects, but we will have to fake it this time.
Include this code snippet in the js/main.js
.
$(document).ready(function() { setTimeout(function(){ $('body').addClass('loaded'); $('h1').css('color','#222222'); }, 3000); });
That will set the body class to .loaded
after 3 seconds and also change the color of our h1
to dark grey.
If you want to learn how to implement imagesLoaded into your project, check out the Simple One Page Template With A Preloading Screen tutorial.
7. No JavaScript, no worries
To ensure that our content is still accessible even without JavaScript being turned on, we can also include the .no-js
fallback.
.no-js #loader-wrapper { display: none; } .no-js h1 { color: #222222; }
And that’s it, you’ve made it!
You’ve created a nice animated preloading screen using CSS3 animations and transitions.
Well done!
Conclusion
What do you think about this simple preloading transition?
Have you seen a cool preloading animation sequence on other sites? Let me know in the comments.
Hello,
I like your loader but it doesn’t work for me. Even the complete package doesn’t work. The loader just doesn’t give the body a class of loaded. I have to insert the class manually to let it work.
Help me plz
Hi Ibo, thanks for the comment. Check the web developer console for any javascript errors. The demo and the downloadable files work fine for me.
Yes, This is working…
thanks !
Try this JavaScript:
document.getElementById(“element”).className = “loaded”;
The webdeveloper toolbar says: ‘ReferenceError: $ is not defined in the rule $(document).ready(function(). When I try your files it gives a lot of css errors. However the javascript error doesn’t show up but that’s because the main.js file isn’t linked.
Ibo, sorry that you still having some issues. You need to link that `main.js` file because it has the code necessary for adding the new body class. That’s why you are getting the js error in your console.
Hope that helps.
No, unfortunately it didn’t help. I used the main.js file provided in the download on this site. I still get the error ‘ReferenceError: $ is not defined’.
Just to clarify, is the same issue happening on my demo site?
Have you included the main.js after a jquery reference?
Thanks, that was the problem. Had to move the thanks for your help!
you just need to load jquery.js with in your html before calling the .js
Hello Petr,
how can i integrate the images loaded plugin?
i tried this:
imagesLoaded(function(){
$(‘body’).addClass(‘loaded’);
$(‘h1’).css(‘color’,’#222222′);
}
the plugin imagesloaded.js is linked correctly
nothing happened
Thanks
Hey Petr!
Love this preloader. Works amazing. I have noticed only one issue. The class .entry-title doesn’t stay near the top of the screen. If you’re half way down the page and hit reload, it’s sitting at the top of the website, not the screen.
Thank you,
Jon
BRILLIANT – Great piece of work and a well structured tutorial.
Im sure your aware of this already Petr, but a quick enhancement for revealing the content on page load, rather than your 3 second delay, try just using…
OR Jquery
WOW! this works so good! It stops doing the loading thing when the page is done loading, Not after 3 sec! and it is sooo simple! Thank you so much. Great tutorial too!
Can not get this to work. Tried both jquery and javascript but nothing.
Hi Alan, can you be more specific what doesn’t work? Do the demo files work on your computer? Check the browser console for any javascript errors.
He mentioned this in the text that because his site is not too heavy so it wont take any time to load and as a result we won’t be able to see the loader. That is why he added a delay of 3 sec so that we could see the loader.
Hope it clears you out…
Hi.I can’t download source.send error !!! please check
Hey Jack, can you clarify what issue you’re having with downloading the files? It works fine for me.
Hi; great article!!!
@Jon just add
to the
.entry-title
class.Thanks for the suggestion for Jon. Cheers
Great HTML CSS tutorial, thank you for making it.
I was able to follow along perfectly until I got to the javascript. I do not write js and I’m having trouble figuring out how to implement the js to add a body class when all the images are loaded.
Right now I’ve got your simple timed script in place and functioning properly. Now, can I simply add the image load detection functionality?
The resources you suggested of imagesloaded and pxloader seem so verbose for the simple functionality I require. And, what is packaged javascript anyhow (I sorta get it, but I’m not a js developer soooo not really)?
Could you please show how to implement your preloader for a typical image rich single paged site, with an unpackaged version of imagesloaded.
TIA
Hello It is nice tutorial.
I have a question i wanna use this preloading screen in my wordpress website. so how can i use it in my wordpress website before loading page. Thanks in advance.
Hi Petr Tichy
Great Tutorial!
And so simple!
Less is more!
Thank you for your effort to teach code!
cheers
Thanks for the feedback Tiago, that’s what keeps me going. Cheers
Hi Petr,
I really love this preloader. Just downloaded your files, paste it into my own site and it works amazing.
However, i’m still missing one thing and that is a image or text in the middle of the circle while its loading. It does not have to rotate, spin or anything.
In this case i want to have a logo to be shown while the site is loading.
My skill level is not high enough to get this to work.
Can you help me out please?
Many thanks in advance and greatings from the Netherlands,
Martin
Hey Martin, thanks for the feedback.
You would need to add another span element inside of the
#loader-wrapper
.It’s not hard, give it a try or you will need use the pre-loader as is.
Cheers
Petr
Hi Petr,
your example here ist great. I worked the half day to get it running. In the end I made some mistake by linking the “main.js” 🙂
What I don’t understand is, that my construction doesn’t run under WIN7 IE 12.0! I tested your demo-site and it runs …
Safari on my MAC, Firefox, Chrome … all fine.
And the animation of the overlay doesn’t fade in the horizontal direction like your demo (when I switch the “transform: translateY(-100%);” into “X” the wipe out doesn’ start from the center of the page …).
I checked all the css and html scripts with your tutorial data. Looks really the same. Have you any idea?
Thanx for a short help 🙂
Cheers, Dirk
Hi Dirk, hard to say without looking at your code. Looks like you might still have some js or css issue with your code.
The best would be to create your preloader from scratch based on the learnings from this tutorial. That way you would know exactly how it’s all put together.
Cheers
Petr
Hello,
Nice tutorial, thanks (:
Thanks Kristijan
Petr,
Great tutorial. Easy to follow and I learned a lot. How would I use this in conjunction with an AJAX request (i.e., not performed for 3 seconds, but during the server request)?
Hi Taylor, thanks for the feedback.
You can simply add a class
.loaded
to thebody
when the AJAX request is completed, that will move the preloader out of the view.And remove the 3sec timeout from my example.
Hope that helps.
Thanks! But how do I add a custom background? I tried all ways but it just does not load my background after the preloader. Thanks.
thank you this is awesome!
Hello Petr,
Thanks for posting this awesome code!
There seems to be a problem with the loader in IE11 (checked it on the demo page).
The loader is flickering and the stripes move few pixels to the side while spinning.
Have you encountered this problem? I’d really appreciate your help 🙂
Hi Tomer, I’ve checked the demo in IE11 and couldn’t see any issues 🙁 Maybe setup a CodePen so we can have a look.
Awesome tutorial Worked perfectly for me
The problem is that how to add some custom text on the loader overlay.
Such as loading document or initializing. What styles i have to use.
I was wondering that our loader is having 3 arcs or semi circles.
can we have 4 or 5 semi circles or arcs
Thank You,
Utkarsh
Wow…
It’s Really Great!
Thanks Jayesh, I am glad you’ve found it useful. Cheers
Same for me. I can’t make it work in IE11. 🙁
What’s the issue with IE11? My demo works perfectly fine in that browser. Check your browser console for any js errors.
Hey Petr!
That was really awesome. I did some modifications to the loader and created it for my site www.ideageek.net. But I would like the loader wrapper to open like a hexagon instead of left and right. Can you suggest me some ideas?
Thanks again for your tutorial 🙂
What changes i have to make if i am using it in my phonegap application for android because when i am using it the different colors lines are appearing not as in demo shown above
still not sure what i am doing wrong. can i get a gif to load in the middle of the page before it goes away? all i got is a white page and changing the body class=loaded isnt working.
pittsstoppcrepair.com
Hi, Thanks ! Every things seems to be ok but it’s impossible for me to get the transition. If i forced “loaded class” in , that’s work but not without. The circle turn and turn and my page never be shown. Any idea ?
Great work…
Great work!! It works perfect but i would like to add more time to the loading page. The moving circle with the black backroung i would like to stay in the page for 5sec and then proceed to the white one. Can you help me?
Hi Marios, just change the duration of the
setTimeout
function to5000
instead of3000. Hope that helps.I have tried it but the time remain the same.. Any idea why this is not changing?
Try refreshing your cache and check.
How can I do this so it is a cookie that only first time visitors see?
Hi Chuy, you would need to create the cookie on the first visit and then add an if statement checking for the cookie.
Hi! Looks great! However I’m a beginner and would like to know how to add this to my wordpress site?
Hi, Petr!
How to show a text under the three lines / circles displaying, ‘Page Loading, please wait …’ in the Loading Screen?
Hi Petr,
This preloading screen looks fantastic. However I’m a beginner and would like to know how to easily 😉 add this to my wordpress site. Thank you!!
Hi Peter, thanks for the suggestion I might create a tutorial on this in the future.
In the mean time, maybe someone else implemented a similar preoloading screen in a WP theme?
Yep, I implemented this feature in my WP site and it’s quite easy. You just need to add the css, js and then include the in the of your index.php file.
this is how it came to me, but I used a different preloader, yet with the help from your code
http://ideageek.net/blog/
hi, ive implement all your methods, but it seems still not quite enough, the svg is plain at first, then overlapping with my site. u can see the problem in www.vkarrendi.work
are you/all got the solution for this?
Hello Petr!
Learnt A Lot From Your Article , Keep It UP. 🙂
I needed a Help ,
Please can you tell me How can I use your PreLoader for my WordPress Website ?
hi Petr!
it’s work and good. but doesn’t work with : “rtl”
what wrong ?
Hi, nice pre loader, is there any way to replace “content” div with any other name, cuz i have content assign to different places, which i cannot change now. if i use “wrapper” instead of “content”, what are the places need to change.
Hi Roshan, I don’t know from top of my head, but you can search and replace all references to “content” in the whole project (html, css, js files). All code editors have this functionality.
Hi,
It’s a very nice preloader!
But is there a way to do this with a Joomla page?
Kind Regards
Hey Mauritz, thanks for the feedback. I am sure there is a way how to implement into Joomla, but I am not very familiar with it. Maybe someone else can point you to the right direction?
Hi Petr,
Great tutorial. I made my own loader using above mentioned techniques and it is working perfect but i have a problem. I am using ajax to fetch data and in many conditions, it takes time and the user sees no response till then. I want that when a user clicks a button to fetch some data from server and data is taking more than a second then it should show loader till the data has been fetched from the server. I’ve no idea how to achieve this. Please help.
Thanks!!
Hi Petr,
Thanks for such an amazing tutorial. Can you post the code required (using jquery) so that the preloader loads only once until the cache/session is cleared. It would be very helpful with users who have multiple tabs in their navbar if the preloader doesn’t load each and every time the visitor visits the index document.
Thank you
Kind Regards
Hi Steve, I would use cookies to manage that.
Here’s a simple article that could help you to get started with cookies:
Working with Cookies in jQuery
Hope that helps.
Great Post !
Hi Petr.
Thanks for this great tutorial. I have implemented it successfully on one of my websites,
I am planning to implement on a new website I am working on but I will like to replace the spinner with another type of animation.
Is this possible and which section of the code needs to change? I am not a developer but with a good and clear instruction, I can figure things out.
Thanks.
Thank you so much for this 🙂 I am a student in HighSchool and i am in a program where we are learning how to code. This helped me SO much. I did nnot understand the JavaScript and could not get the class=loaded to be added to the body but otherwise this was Amazing.
Thanks!!!!
Thanks Cameron, I am glad the tutorial was so useful. Good luck with your studies. Cheers Petr
Thanks dude, great piece of work!
It works very well for me!
No probs Nacho, glad to hear that it workwd for you. Cheers Petr
Hi,
Thank you for this great preloader!
I wonder to know how I could set the preloader only once by using a coockie. The reason is that i’m using it as a splash page.
Thank you for your help,
This preloading screen increases window height unexpectedly, I guess there is something in css which increases whole window height to more than 1500px even on the mobile screen of 568 max height. I used jQuery(window).height() to get window height.
Just awesome! Thanks for this great preloader tutorial, I made it work in less then 10 min.
I had some ugly loading going on, as I was working with a greensock animation which layered all my animated text on top of eachother when entering the page, as the javascript was loading after the page content.
Thanks a mill!
Sabrina
you can see it in action on www.useradventure.com
Hi,
Thanks for making this tutorial. There are not many resources which walk you through like this one does. Great job!
Dude you are the bombskie! Thanks a lot for this tutorial. You totally rock!!
You’re welcome Will, thanks for stopping by.
Nice tutorial. Preloader looks nice. I wanna include this in my website. But don’t know you allow this or not. Can I include it?
No problem Abid, use it for whatever you like. Cheers
No problem, use it for whatever you like Abid. Cheers
Me and my pals Love this Pre Loader Effect. Hands down the best out there!
Our quick question for you is this;
How would we get it to fade in the page instead of the sliding out effect?
Thanks!
Hi Brian,
a quick suggestion would be to remove
transform: translateX
from left/right sections and only animate opacity and visibility. In fact you don’t need two parts but only one preloader container fading out.Hey Petr, it’s really awesome. I successfully added it to my side, but the wheel won’t stop. I am not quite sure what I am doing wrong. Do you have any suggestionst?
Cheers
Hi Katrin, there is a 3 seconds “manual” delay in my demo. Can you check your browser console for any JS errors?
I had some problems with main.css
#loader doesn’t really disappear, it continues but invisible,
I just add ‘display: none;’ :
.loaded #loader {
/* opacity: 0;*/
display: none;
-webkit-transition: all 0.3s ease-out;
transition: all 0.3s ease-out;
}
Hey Carlos, sounds like you had some JS error in your code and the
.loading
class wasn’t removed. Check the browser console for any JS error.Thanks Petr!
I didn’t checked js, you solved my problem:) (sorry :/ I was in a hurry and I didn’t read all comments bellow) thanks anyway!
its works for me but, how to add more circle?
You would have to adjust the markup, currently it’s using
border
,:before
and:after
elements. Try to add aspan
inside of the loader and style it accordingly.Thank you very much for this splendid tutorial. I have a question, I’d like to add an icon in the middle of this circular animation (png image). How can I have it in there?
Hi Keith, you can simply apply a
background-image
to the#loader-wrapper
with abackground-position: 50% 50%
, that should do the trick.Hey Thanks Petr for the prompt response. Above block doesn’t work for me.
You are missing
;
after thebackground-image
.Hi,
I really like this! But what would I need to do if I make my own .gif and use it as a preloader?
Thanks
Hi Mauritz, if you want to use your own loading .gif you would need to use a plugin such as PxLoader or ImagesLoaded and hide your gif after the loading is completed. Please refer to the plugins documentation for more details. I would recommend ImagesLoaded if all you are preloading are images or background images.
Is there a way to make ease-in transition in between pages of sites switch?
Mean I put such code on 3 pages, every time i change one page to another the preloader come in instantly, is there a way to delay page change transition, play two sections left and right come back then page changes and animation of preloader can do it deal?
Hi Vitaly, there is always a way to do things. What have you tried to make it work the way you want?
I tried add such java code to main.js
then add class=”transition_to” to my “href” links so this make preloader appear on screen when I click other page links and after delay page just get changed to another one, which also contains preloader code.
But it’s look ugly, problem is that i want to that left//right section appear slowly from both sides to screen center then preloader wheel fadeout, and only then page get changed and it continue it’s job, except that it’s first page launch, where it’s appear instantly as it done now.
So the idea is something like play that preloader animation vice versa, maybe you can help me to do that, my java skills is very low.
Also my solution have problem with white flashes in google chrome, guess that white screen blinks appear where page get changed to other one.
Hi! This was very helpful!! Got it working right away! However, I have two questions: First, I’m using bootstrap and can’t seem to get my navbar (using fixed top) to be hidden until after the animation finished. Do you have any tips on that? Also, I was able to get the image in the middle of the preloader, but I’m curious, what would it take to rotate the image? Again, this was a great tutorial! I am working on my very first Rails project and I was surprised at the ease I was able to implement this! Thank you!
Hey Joey, I am not using Bootstrap, but check their documentation, I am sure there is a class to make the nav hidden by default.
If you add the background image to the elements with the
spin
animation, the image should rotate automatically.Good tutorial.. Good article..
Hi, Petr! Your loader is great, but what if I want to add many round lines on the loader, what would be the `animation-duration` of each line?
– Thanks, in advance!
Hi Petr, my loader does not go away when it is fully loaded.
How can I fix this problem?
Thank you!
Check your browser console for any JS errors.
Hi Petr,
your preloader looks and works nice. Thank you !!
Do you have a code snippet to implement your preloader for an existing website ?
The idea :
Instead to load your Demo Content, after the screen animation, it would be nice to open the existing website… is that possible ?
Cheers
Tom
Hi Tom, no I don’t have a code snippet that would make it work with your existing site. You will need to implement it manually. Cheers
Hi Petr, I like a lot your loader, but if I put the loaded class in the body it wont work, and with the main.js only works for setting a time that the loader will be visible but not to make the loader stay until the page loads.
I removed the main.js from the page and added this code:
$(window).load(function(){
$(“#loader-wrapper”).fadeOut(“slow”);
});
It works to show the loader until the page is load but the curtain effect wont work.
Hi Daniel, hard to say what could be the issue, check the browser console for any errors.
This worked out really well for me and thought … I need text too. Here’s the additions for anyone interested.
Thank you!! What a great tutorial!!! congratulations mate!
😀
Hi there,
It looks pretty nice, but there is one problem.
I did everythink like in the tutorial, but the loading think don’t stop, it continue loading the page, can you please help me with this.
Now that ive made it, how do i then add it to my site?
WOW! this works so good! It stops doing the loading thing when the page is done loading, Not after 3 sec! and it is sooo simple! Thank you so much. Great tutorial too!
Muito bom! =) Parabéns!!!
Hi,
I am looking for a preloader jquery code in which, image is split from every corner and joint.
Thanks in advance
You are a boss, Petr. Thank you!
You’re welcome Steven.
This is very nice, but i have a question. How can we lock the page so the user is not able to scroll while the page is loading? As you see, in the example you provide, while the page is loading, you can scroll it. And when the page is done loading and the loader fades away, you’re already at the bottom of the page. How can we prevent that and use some kind of disableScroll function while the loader is active?
Thank you!
Hey Petr,
Great tutorial!! Any hints on how to get it working for mobile? Thanks for the lesson.
Perfect loading code, thakns
This is awesome. I learned alot with this. How do you put a loading bar though?
Hello Petr Tichy!
Thank you for this tutorial, very helping!
Can we have a webpage to pre-load before the main page loads ?
Hi! First of all, great post!
I’m having issues adding this loading screen to my WordPress site. Do I need to have any extra considerations when doing this on a WordPress site? It seems like the JQuery timeout is not working for me, like you can see on my testing site:
https://pruebasweb.sectorhosting.com/
I hope you can help me sort this out…
Thanks!
everything should work, i cant see from your site but im guessing you need to add this code to your page header. you should learn html5 instead of wordpress.
Everything is working, but I was trying to make the overlay semi transparent instead of solid color #222, couldn’t get it to work by setting opacity like below:
#loader-wrapper .loader-section {
position: fixed;
top: 0;
width: 51%;
height: 100%;
background-color: #222;
opacity: 0.8;
…
Can you help?
This really helpful!
Thanks
Really great Preloader and instructions.
Is there a way to cange the colours of the spinning loader to match peoples company colours? Its a branding thing. Thanks in advance.