Page transitions are a thoroughly popular tool to help make your website stand out!
Smooth, fluid, and creative transitions often sets awarding winning websites apart from the rest.
In this tutorial we will demonstrate how to create a simple page transition using Barba.js and GreenSock.
What you will learn:
- How to setup Barba.js
- How to create animated page transition using GreenSock
- How to use Barba.js Hooks
Introduction to Page Transitions
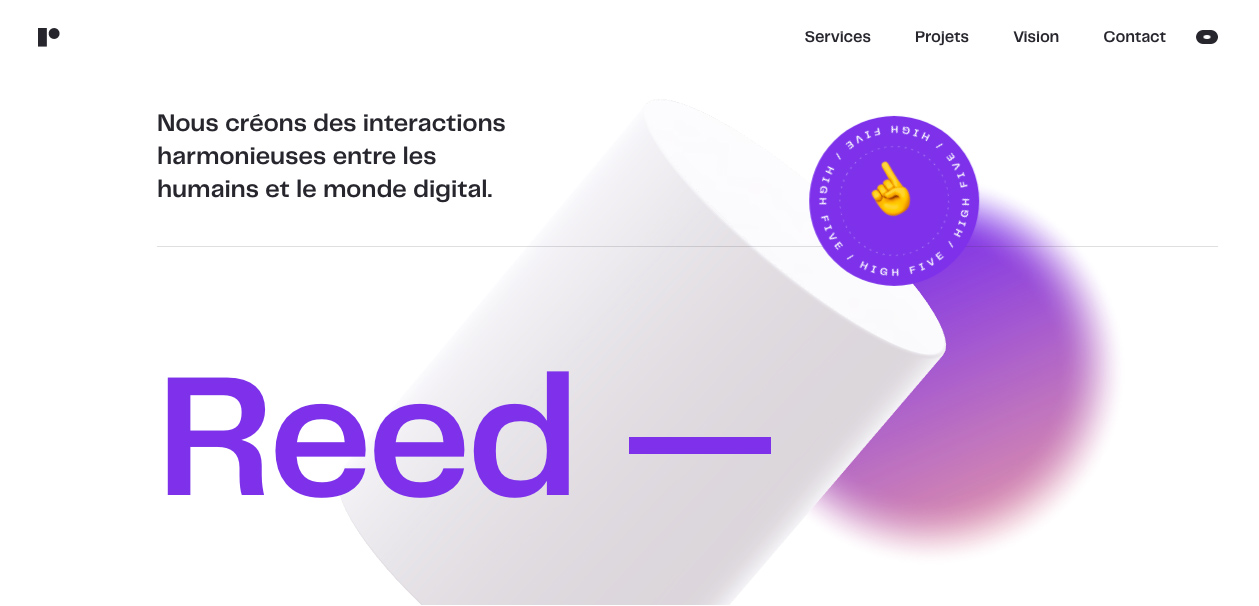
Whilst creating page transitions may sound difficult, Barba.js makes the process incredibly easy.
There are two types of page transitions:
- Page transitions with a take over or loading screen – eg. https://viens-la.com/
- Seamless page transitions – eg. https://www.reed.be/
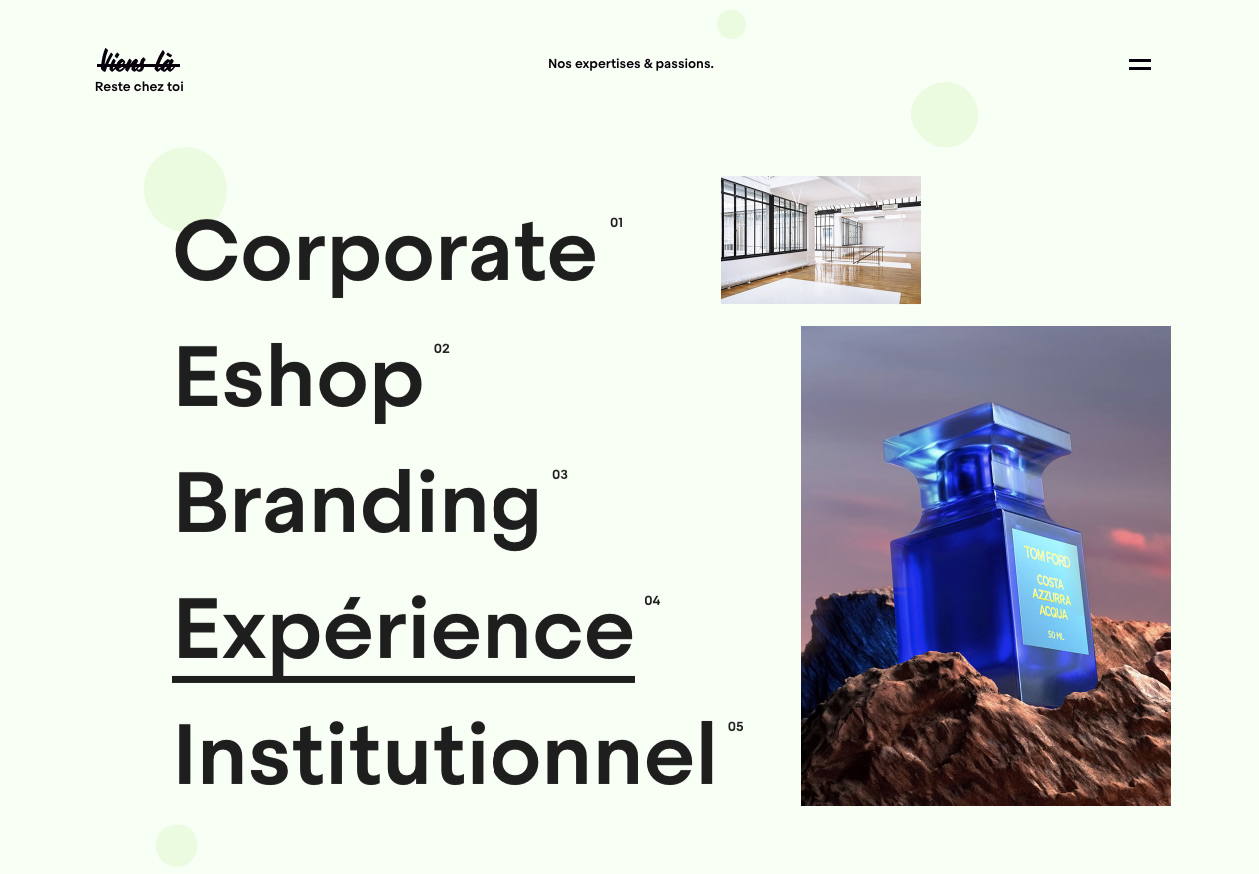
Now, let’s walk through the process of creating a loading screen page transition on a project with 2 static html pages.
/css/app.css /js/main.js index.html page2.html
1. Create a loading screen
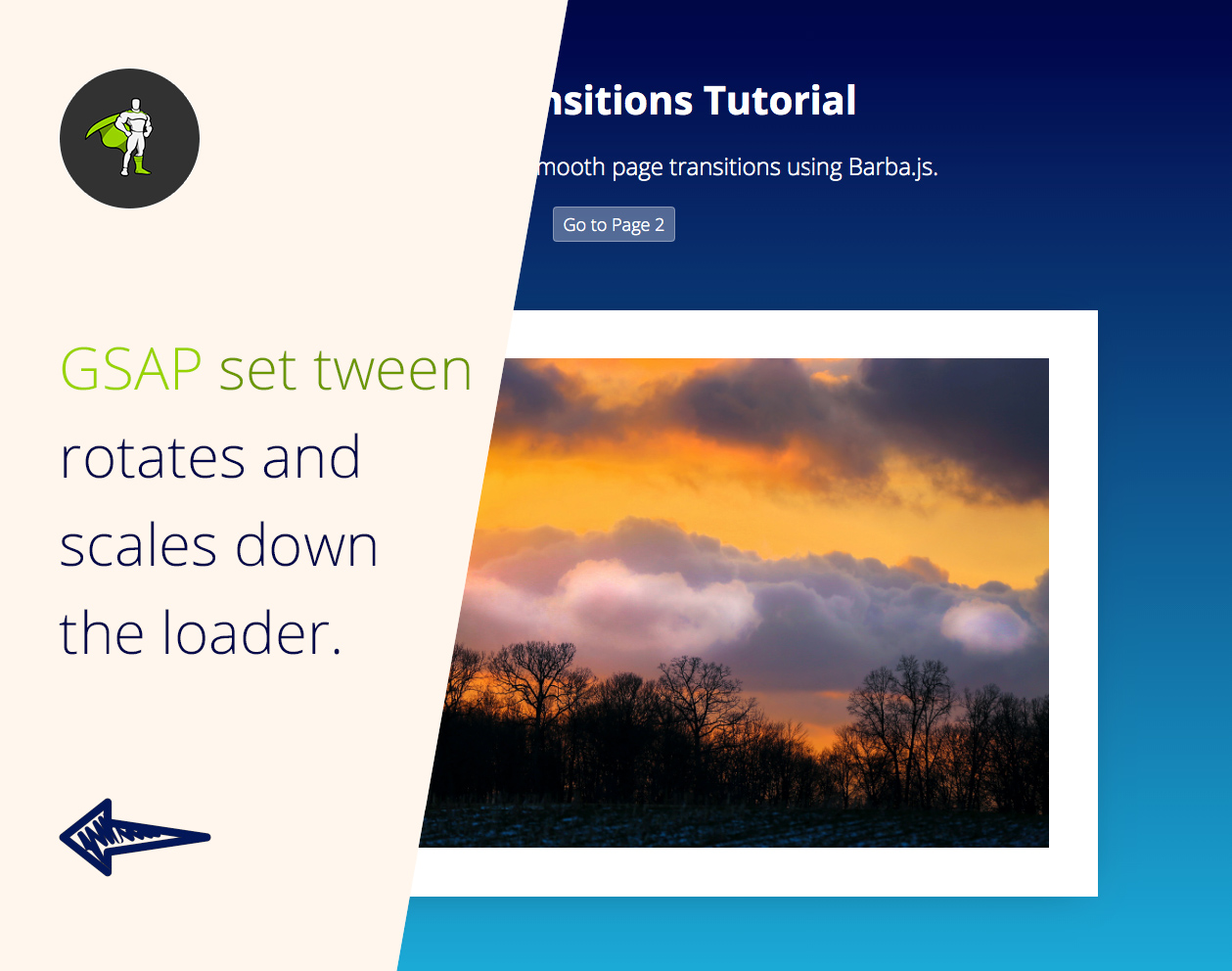
Firstly, we will create a loading screen .loader
. This will be a container that will cover our screen while Barba.js is loading the next page.
<div class="loader"></div> .loader { position: fixed; width: 100vw; height: 200vh; pointer-events: none; background-color: #FFF6ED; z-index: 1; visibility: hidden; opacity: 0; }
We will make it position: fixed
and the height twice as tall as the screen height: 200vh
. This is because we will soon be slightly rotating it. Without any rotation a height: 100vh
would be enough to cover the whole screen.
Opacity
and visiblity
are set to 0
and hidden
, because we don’t want to see a flash of the loader before GSAP scales it down.
// reset position of the loading screen gsap.set(loader, { scaleX: 0, rotation: 10, xPercent: -5, yPercent: -50, transformOrigin: 'left center', autoAlpha: 1 });
In the main.js
we are rotating the loader and setting the scaleX
to 0
to make it invisible for the user. At the same time we are setting visibility: visible
and opacity: 1
using GreenSock’s handy autoAlpha
property.
2. Include Barba.js

Include Barba.js at the bottom of both HTML files.
<script src="https://unpkg.com/@barba/core"></script> <script src="https://cdnjs.cloudflare.com/ajax/libs/gsap/3.3.4/gsap.min.js"></script> <script src="js/main.js" defer></script>
I am also including GreenSock because Barba.js is not an animation library – it only takes care of content loading.
Your preferred animation library and style of page transition is completely up to you.
I tend to choose GreenSock for its flexibility and easy functionality. I have also become very familiar with it.
If you want to learn how to use GreenSock from scratch, check out GreenSock 101.
There are plenty of other animation libraries that would also work well, such as popmotion, animejs, mojs or spirit.
3. HTML Markup
Barba.js requires two special data-attributes somewhere in your markup.
<body data-barba="wrapper"> <!-- content that stays the same on all pages - eg. header --> <div id="intro" data-barba="container"> <!-- content that will change from page to page --> </div> <!-- content that stays the same on all pages - eg. footer --> </div>
data-barba="wrapper"
specifies the main wrapper of your page structure. This could be in the body
or any other HTML element.
data-barba="container"
is a section of your page which will be “reloaded or updated,” with the incoming content from the other page.
Anything outside of the container
will not change between the page transitions.
You can use any markup you want, the only requirement is that the wrapper
always needs to wrap the container
.
4. Init Barba.js
barba.init({ transitions: [{ async leave() { await loaderIn(); }, enter() { loaderAway(); } }] });
In the main.js
, we then initiate Barba.js to create our transition.
leave()
will be executed first, followed by enter()
.
loaderIn()
is a function that returns GSAP tween which stretches our .loader
to cover the whole screen.
loaderAway()
is a function that returns GSAP tween which scales the loader back to scaleX:0
, when the new page is loaded underneath it.
5. GreenSock Animations
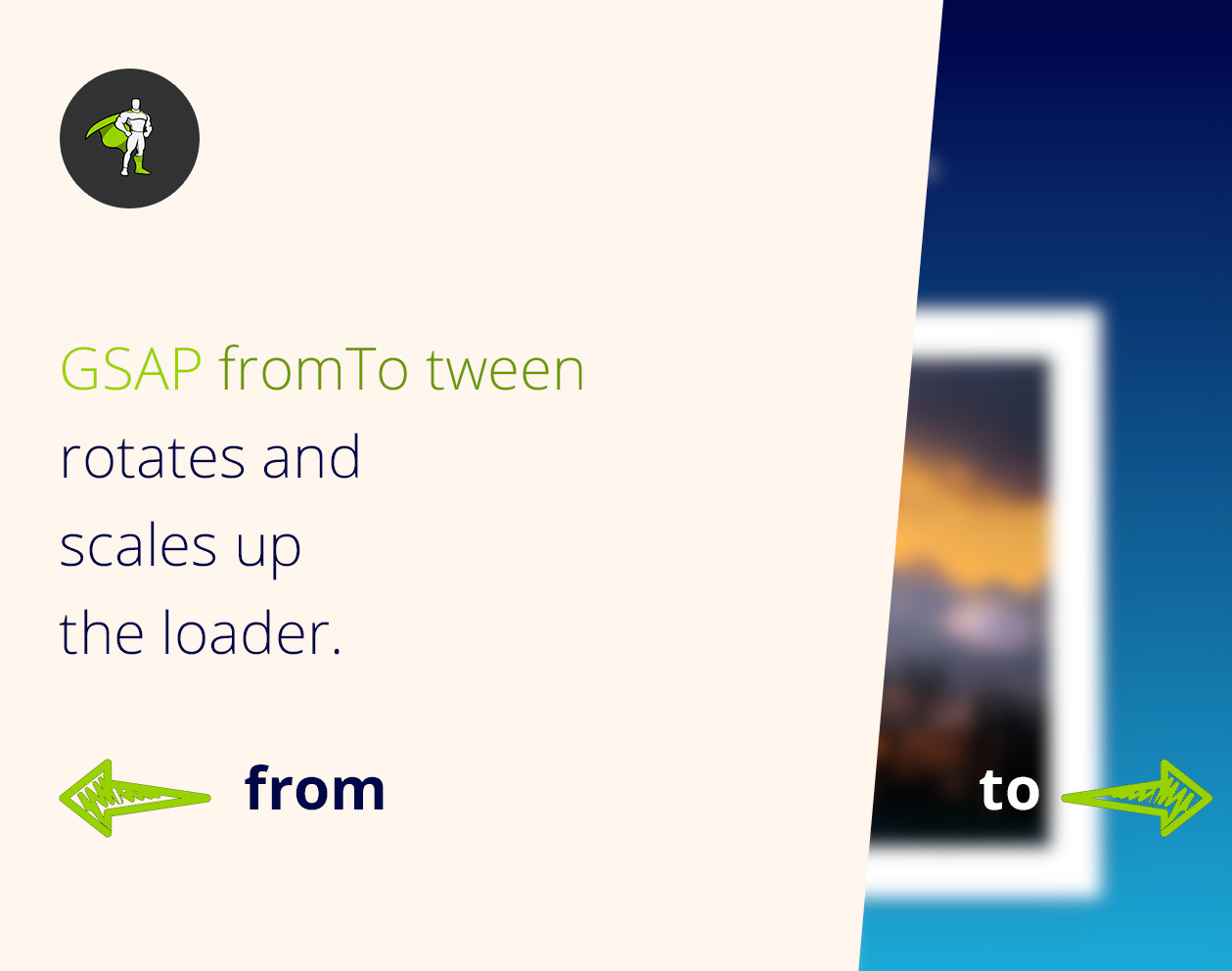
Now let’s have a look at the two tweens which create the transition.
function loaderIn() { // GSAP tween to stretch the loading screen across the whole screen return gsap.fromTo(loader, { rotation: 10, scaleX: 0, xPercent: -5 }, { duration: 0.8, xPercent: 0, scaleX: 1, rotation: 0, ease: 'power4.inOut', transformOrigin: 'left center' }); }
fromTo
tween takes care of the first half
of transitions and covers the screen when it’s completed.
We are setting the transformOrigin
to be 'left center'
. This makes it grow from left to right.
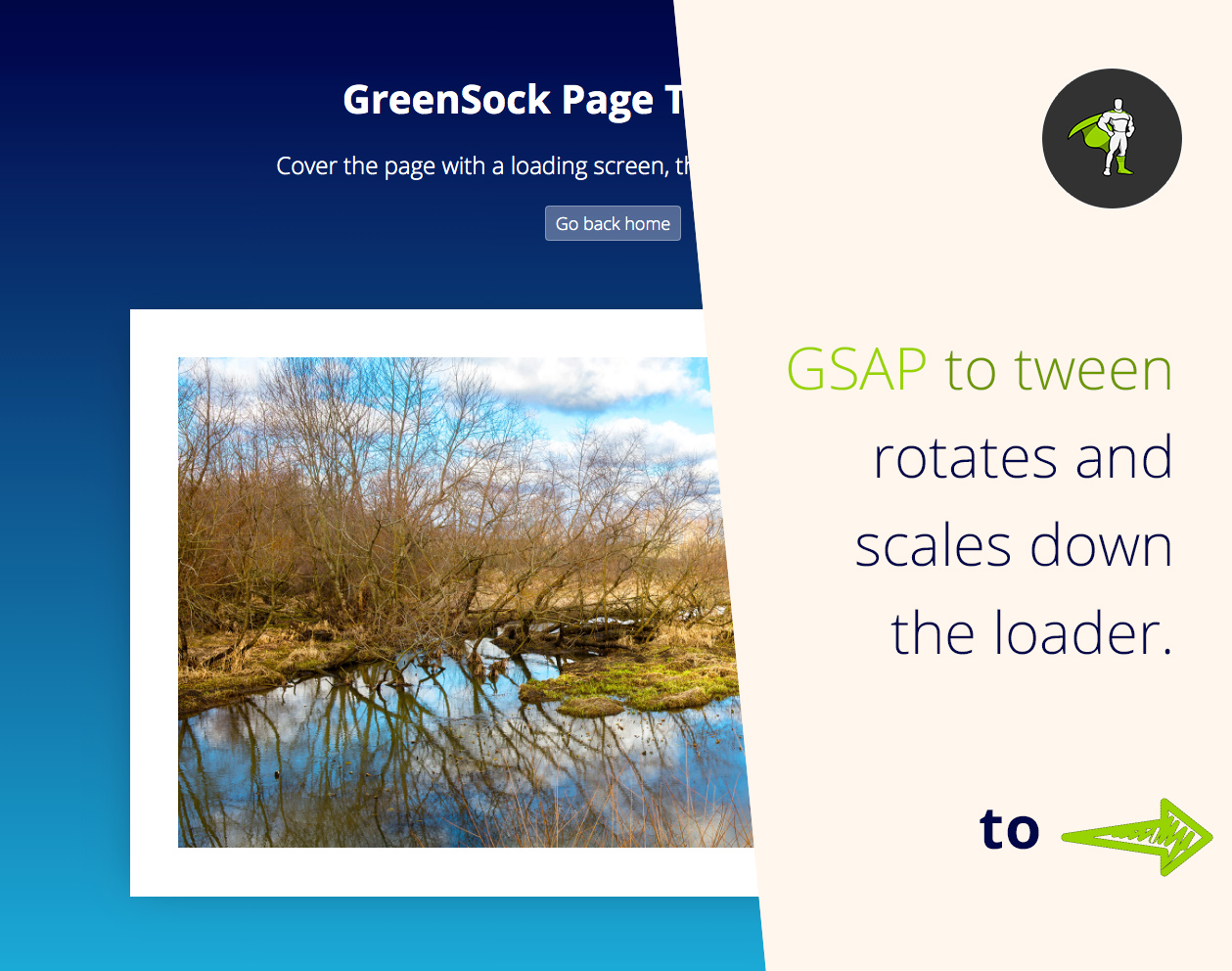
function loaderAway() { // GSAP tween to hide loading screen return gsap.to(loader, { duration: 0.8, scaleX: 0, xPercent: 5, rotation: -10, transformOrigin: 'right center', ease: 'power4.inOut' }); }
This tween reveals the new page which was loaded underneath the loader by Barba.js.
By changing the transformOrigin
to 'right center'
we create the effect of the loader disappearing on the right side of the screen.
6. Before and after the transition
Barba.js also gives you access to specific lifecycle methods or hooks that you can tap into.
One common example would be to add css class to your page to prevent users from double clicking on links.
// do something before the transition starts barba.hooks.before(() => { document.querySelector('html').classList.add('is-transitioning'); }); // do something after the transition finishes barba.hooks.after(() => { document.querySelector('html').classList.remove('is-transitioning'); });
.is-transitioning { pointer-events: none; cursor: progress; }
Another example would be to enable scrolling to the top of the newly loaded page. We can use .enter
hook for that.
// scroll to the top of the page barba.hooks.enter(() => { window.scrollTo(0, 0); });
Final Demo
Related Resources
- Page Transitions Tutorial – Barba.js and GreenSock – Part 2
- Practical GreenSock – Premium Online Tutorials
Conclusion
This may have been just a basic example, however I hope you can now see implementing page transitions can be a simple task!
The ultimate key is to master Barba.js and an animation library of your choice. If you’d like to go with GreenSock, learn everything you’ll need to know at GreenSock 101.
Today we really only scratched the surface of page transitions. It is a broad area, with lots of creative options out there.
So, if you have you seen other interesting page transitions you would like to learn about, please let me know! I’d be happy to make more in-depth page transition tutorials.
Hi
This is a great tutorial. We have used Barba.js for a few sites we have built. We find it gives a nice transition between our pages.
We even use it on our own site with GSAP. As well as a custom animation for our 404 page. air66design.com
Thanks David, looks like Barba is quite popular these days. Nice work on your site.
This looks like a great course. Page transitions and animations are so highly desirable by clients now, so it’s great to see a course that marries the two.
Courses are showing as closed, why?
Hi Kenneth, I will be launching new courses next week. In the meantime you can enter a giveaway. Old courses are outdated and will not be reopening. Any other questions or tutorial suggestions, please let me know.
Hi! Thanks! I have some troubles.. I’ve seen the demo and it’s working great, but when I download the files it’s not working, even on localhost. Do I need something else?
Thanks!!
What does not work? Are you looking at the files via a localhost server (VSCode Liveserver)? Any console log errors?
Well, it seems that works fine in vscode liveserver. Where it’s not working is in mamp. Why is there any difference? Shouldn’t it just be the same?
Thanks again Petr. Great work
Yes, it should. Not sure how is your mamp setup, maybe it’s because your file in a subfolder?
Hi,
Thank you for sharing this tutorial because I am struggling in understanding the GSAP and Babra.
I have a WordPress website and wanted to implement GSAP and Babra on it. Do you provide any tutorials for this.
Hi Usama, I don’t have any tutorials on Barba and WP.